Laravel Service Classes: A Deep Dive for Developers
- Glorywebs Creatives
- Feb 6
- 3 min read
Laravel, one of the most popular PHP frameworks, offers a structured and efficient way to develop web applications. One of its powerful features is service classes, which help developers maintain a clean and organized codebase. If you're working with Laravel Development Services, understanding how to use service classes properly can enhance the scalability and maintainability of your applications.
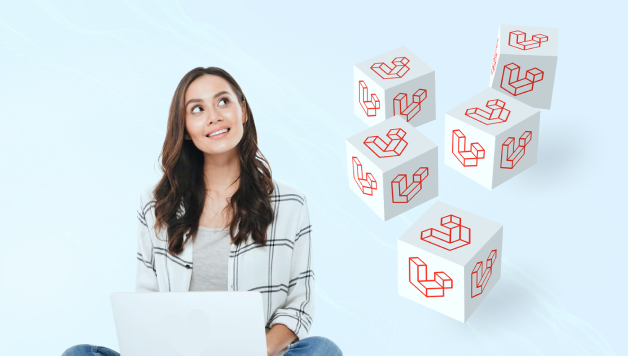
What Are Laravel Service Classes?
Laravel service classes are used to encapsulate business logic separately from controllers and models. This approach follows the Single Responsibility Principle (SRP), making the application more modular and easier to maintain. Laravel also provides a robust Laravel Package ecosystem that can further enhance service classes and streamline business logic.
Instead of keeping complex logic inside controllers, developers can move it to service classes, allowing controllers to focus only on handling HTTP requests and responses.
Why Use Laravel Service Classes?
Using Laravel service classes offers several benefits:
Code Reusability – Service classes allow the same logic to be used across different parts of the application.
Improved Readability – Controllers remain clean and easy to read.
Scalability – Business logic is neatly separated, making future updates and modifications seamless.
Better Testing – Unit testing becomes easier as service classes can be tested in isolation.
Follows SOLID Principles – Service classes support better design patterns and architecture.
How to Create a Laravel Service Class
Step 1: Create the Service Class
Laravel does not have a default command to create service classes, so you need to create a directory and class manually.
Navigate to your Laravel project and create a Services directory inside the app/ folder:
mkdir app/Services
Then, create a new PHP file inside the Services folder. For example, let's create a UserService.php file:
<?php
namespace App\Services;
use App\Models\User;
class UserService
{
public function createUser(array $data)
{
return User::create($data);
}
}
Step 2: Using the Service Class in a Controller
Once the service class is created, you can use it in a controller by injecting it through dependency injection.
Modify your UserController.php:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Services\UserService;
class UserController extends Controller
{
protected $userService;
public function __construct(UserService $userService)
{
$this->userService = $userService;
}
public function store(Request $request)
{
$user = $this->userService->createUser($request->all());
return response()->json($user);
}
}
Step 3: Registering the Service Class (Optional)
You may register the service class as a singleton in the AppServiceProvider for better control over dependency injection:
public function register()
{
$this->app->singleton(UserService::class, function ($app) {
return new UserService();
});
}
Latest Laravel Updates on Service Classes (Laravel 10 & Beyond)
With the latest Laravel versions (Laravel 10+), there have been some enhancements that indirectly improve the way service classes work:
Invokable Controllers – You can now define single-action controllers, reducing the need for extra boilerplate code when using service classes.
Improved Dependency Injection – Laravel now has better support for injecting services into middleware, event listeners, and even console commands.
Closure-Based Service Binding – The ability to bind services dynamically using closure-based service binding in the service container.
Best Practices for Laravel Service Classes
To ensure optimal use of service classes, follow these best practices:
Keep them focused – Each service class should handle only one aspect of business logic.
Avoid storing state – Service classes should remain stateless to ensure they work well with Laravel’s dependency injection.
Use dependency injection – Instead of creating new instances within the service, inject dependencies via constructors.
Keep controllers thin – Move as much business logic as possible to service classes.
Conclusion
Laravel service classes offer a clean and modular way to structure your business logic, improving maintainability and scalability. As Laravel continues to evolve, features like enhanced dependency injection and improved service binding make service classes even more helpful. You can develop robust applications that follow best practices and maintain a structured architecture by implementing service classes properly.
Comments