Beginner’s Guide to React Design Pattern for Developers
- Glorywebs Creatives
- Feb 10
- 3 min read
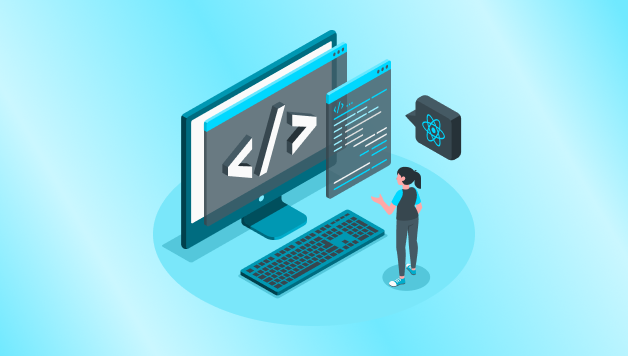
React is a powerful JavaScript library used to build user interfaces, and it’s known for its flexibility and reusable components. But to become proficient in React, developers need to understand React Design Patterns. These patterns provide efficient ways to structure and organize React components, making your codebase easier to manage and scale. In this beginner’s guide, we’ll explore key Design of React Patterns and how you can apply them to your React projects.
What Are React Design Patterns?
In simple terms, Design patterns in React are reusable solutions to common problems faced during React development. These patterns help developers solve issues such as component organization, state management, and performance optimization.
As a React Developer, you will encounter different patterns that fit various use cases. By understanding these patterns, you can improve code readability, maintainability, and consistency across your projects.
Why Should Developers Learn the Pattern of React Design?
When working with React for developers often face challenges in building scalable and maintainable applications. Using React Design Pattern can help:
Improve Code Organization: Proper patterns help structure your components logically and modularly.
Boost Reusability: Design patterns allow you to reuse code in multiple places, reducing redundancy.
Enhance Performance: Some patterns, like lazy loading and memoization, improve app performance.
Simplify State Management: Effective state management becomes more manageable with specific patterns.
Now, let's dive into the most important Design Patterns React every developer should know.
Popular Patterns of React Design for Beginners
1. Container and Presentational Pattern
This pattern divides components into two types: Container components (or smart components) and Presentational components (or dumb components).
Container components manage state and logic.
Presentational components handle rendering the UI based on the data they receive.
For example, if you are building a to-do list app, the Container component would handle adding, deleting, and updating tasks, while the Presentational component would display those tasks in a list format.
2. Higher-Order Component (HOC)
A Higher-Order Component (HOC) is a function that takes a component and returns a new component with additional props or logic.
HOCs are great for reusing component logic, especially when dealing with common tasks like authentication, logging, or fetching data. For instance, you might have a withAuth HOC that wraps around your main components to enforce user authentication before rendering.
3. Render Props Pattern
The Render Props pattern is used to share code between components using a prop whose value is a function. The function is called during rendering, allowing the component to dynamically adjust its behavior.
For example, you could use a Render Props pattern to handle a modal’s open and close functionality, enabling different components to control the modal state.
4. Compound Component Pattern
The Compound Component pattern is useful when building components that need to work together. It’s especially helpful in cases where parent and child components need to share state and behavior.
In a Compound Component structure, the parent component exposes APIs for the child components to interact with, which keeps the component logic unified and cohesive.
5. State Reducer Pattern
The State Reducer pattern gives you more control over the state logic of a component by allowing you to define a reducer function that processes the component’s state updates.
This pattern is particularly useful when you need more flexibility than React’s built-in state management provides, allowing for custom actions and transitions.
React Patterns for Beginners: Key Takeaways
As a beginner in React development, learning these design patterns in react will enable you to create scalable, maintainable, and reusable code. Here’s a quick recap of the most useful patterns:
Container and Presentational Pattern: Separate logic from UI rendering.
Higher-Order Components (HOCs): Enhance components by adding functionality.
Render Props: Share code dynamically between components.
Compound Components: Group related components that work together.
State Reducers: Customize state management for more control.
Conclusion: Embrace React Design Pattern for Cleaner Code
Mastering design patterns in React for developers who want to build efficient, reusable, and scalable React applications. By understanding and implementing these patterns, you’ll be able to solve common development challenges with ease. So, take some time to experiment with these patterns and see how they can enhance your React projects!
Comments